Arduino-Based Temperature Automated Curtain Railing System
✅ Paper Type: Free Essay | ✅ Subject: Engineering |
✅ Wordcount: 1162 words | ✅ Published: 31 Aug 2017 |
CHAPTER 5
THE SOLUTION
5.1 Overview
The goal of Arduino-Based Temperature Automated Curtain Railing System is to ease the daily operation of residential curtains by automating the curtains where the curtains doesn’t require human effort and operates by using electric powered motors. Based on the implementation methods discussed in the previous chapter, the proposed solution to achieve the goal and objectives is thoroughly explained and evaluated in this chapter. Arduino-Based Temperature Automated Curtain Railing System has two methods of application. A temperature module sensor is integrated into the system to read and send temperature data which then the systems evaluates and decides in closing and opening the curtains. Also, a Bluetooth module is added to give the system the capability to be connected and controlled by users through a smart phone. For this application, an existing Android app is used to establish the connection between the smartphone and the Arduino microcontroller. The prime language used in this system is C++.
5.2 Arduino Microcontroller
#include #define btRX 3 // BT RX pin
#define btTX 4 // BT TX pin
#define ledClose 9 // Close indicator LED
#define ledOpen 10 // Open indicator LED
#define swClose 11 // Curtain Close limit switch input
#define swOpen 12 // Curtain Open limit switch input
#define ledOn 13 // Power ON and RECV indicator LED
#define LM35pin A0 // LM35 temp pin
#define motRev A5 // Motor Reverse control pin
#define motFor A4 // Motor Forward control pin
|
Figure 5.1: Declaring the pins
The microcontroller pins are first declared. Each module is assigned to a pin. The declared value is used later on throughout the code instead of the pins.
5.3 Initializing System Components
void measTemp() { reading = analogRead(LM35pin); // Read sensor tempC = reading / 9.31; // Convert to Celcius delay(100); } |
Figure 5.2: Initialize LM35 Temperature sensor
System reads the value produced from the LM35 pin. The value produced by the sensor is in voltage metric and is converted to Celsius. The parameter delay is set to 0.1 seconds.
measTemp(); // Take a temperature reading if (HC05.available()>0) { char inByte = HC05.read(); delay(100); |
Figure 5.3: Initialize HC-05 Bluetooth module
Bluetooth module reads the temperature data from temperature sensor and sends the data to any smartphone device connected through Bluetooth. The parameter delay is set to 0.1 seconds.
void blinkLED() { digitalWrite(ledOn, LOW); delay(50); digitalWrite(ledOn, HIGH); delay(50); } |
Figure 5.4: Initialize LED indicator
LED is set to blink by turning on and off for 0.05 second intervals. This is used later on as to indicate the system is running.
5.3 Curtain Initializing Functions
void chkStatus() { openStat = digitalRead(swOpen); closeStat = digitalRead(swClose); if (openStat == HIGH && closeStat == LOW) // Curtain is open { digitalWrite(ledOpen, HIGH); digitalWrite(ledClose, LOW); HC05.println(“Curtain OPEN”); } else if (openStat == LOW && closeStat == HIGH) // Curtain is closed { digitalWrite(ledOpen, LOW); digitalWrite(ledClose, HIGH); HC05.println(“Curtain CLOSED”); } else if (openStat == LOW && closeStat == LOW) // Curtain state not known { // so close it first HC05.println(“Initialise…..”); delay(500); HC05.println(“Waiting to CLOSE”); //displays this while curtain closing { blinkLED(); closeStat = digitalRead(swClose); digitalWrite(motRev, HIGH); digitalWrite(motFor, LOW); }while (closeStat == LOW); //Wait until Close Sw = HIGH digitalWrite(motRev, LOW); digitalWrite(ledOpen, LOW); digitalWrite(ledClose, HIGH); HC05.println(“Curtain CLOSED”); } |
Figure 5.5: Function to initialize the curtain
The curtain functions are presented above. The function detects if the open switch is engaged and the close switch is not. If it’s true, then red LED is switched on indicating the curtain is open and “Curtain OPEN” character is sent to the Bluetooth module which later sends to any connected smartphone. Else if, the yellow LED is switched on indicating curtain is closed and “Curtain CLOSE” character is displayed on smartphone. Else if the function is unable to read the switch or if the curtain state is unknown, the curtain is closed first by default and only then system begins its function. “Waiting to CLOSE” is displayed while curtain is closing.
5.4 AUTO/MANUAL Mode Selection
case ‘3’: // Select Manual Mode on smartphone HC05.println(“Manual ON”); autoStat = 0; break; |
Figure 5.8: MANUAL mode selection
The code above switches the default auto mode to manual, giving access to Bluetooth module to receive and send command from connected smartphones.
“Manual ON” is displayed in smartphone indicating that manual mode has been enabled and ready. The case switch statement here is set as case ‘3’.
case ‘4’: // Select Auto mode on smartphone HC05.println(“Auto ON”); autoStat = 1; break; |
Figure 5.9: AUTO mode selection
The code in Figure 5.6 switches from manual mode to auto, enabling the temperature module to control the whole system automatically based on temperature.
“Auto ON” is displayed in smartphone indicating that auto mode has been enabled and live. The user has no control on the system in this mode, unless select manual mode. The case switch statement here is set as case ‘4’.
5.4 Bluetooth Initializing Functions (MANUAL MODE)
switch (inByte) { case ‘1’: // Open Curtain MANUAL MODE HC05.println(“Waiting to OPEN”); do { blinkLED(); openStat = digitalRead(swOpen); digitalWrite(motFor, HIGH); digitalWrite(motRev, LOW); }while (openStat == LOW); digitalWrite(motFor, LOW); digitalWrite(ledOpen, HIGH); digitalWrite(ledClose, LOW); HC05.println(“Curtain OPEN”); break; |
Figure 5.6: OPEN Curtain Bluetooth Command
The code on top shows the OPEN curtain function through Bluetooth connection. The code also enables the Bluetooth module to send curtain status in real time. Once curtain is fully open, the red LED is switched indicating the curtain is fully open. The green LED blinks, indicating the motor is running and executing. “Curtain OPEN” is displayed on smartphone screen when the curtain has successfully completed the open process. The function above is declared as a case switch statement giving it case ‘1’. The Android application installed in the smartphone recognizes the case ‘1’ function when the command setting for a specific button is set to 1.
case ‘2’: // Close Curtain MANUAL MODE HC05.println(“Waiting to CLOSE”); do { blinkLED(); closeStat = digitalRead(swClose); digitalWrite(motRev, HIGH); digitalWrite(motFor, LOW); }while (closeStat == LOW); //Wait until Close Sw = HIGH digitalWrite(motRev, LOW); digitalWrite(ledOpen, LOW); digitalWrite(ledClose, HIGH); HC05.println(“Curtain CLOSED”); break; |
Figure 5.7: CLOSE Curtain Bluetooth Command
The code on top shows the CLOSE curtain function. Yellow LED is turned on when the curtain is fully closed. As the curtain closes fully, “Curtain CLOSED” is displayed on smartphone screen. The case switch statement here is set as case ‘2’.
5.4 Temperature Sensor Initializing Function (AUTO MODE)
else if (autoStat == 1 && tempC > 32 && openStat == HIGH && openClose == 1) // Temperature exceeds 32 { measTemp(); HC05.println(tempC,1); // Send Temperature data //HC05.println(“Waiting to CLOSE”); //displays this when curtain closing do { blinkLED(); closeStat = digitalRead(swClose); digitalWrite(motRev, HIGH); digitalWrite(motFor, LOW); }while (closeStat == LOW); // Wait until Close Sw = HIGH digitalWrite(motRev, LOW); digitalWrite(ledOpen, LOW); digitalWrite(ledClose, HIGH); HC05.println(“Auto CLOSED”); // display when curtain closed openClose = 0; } |
Figure 5.10: Curtain CLOSE when temperature high
The above code initializes the curtain to close when the temperature exceeds 32-degree Celsius in automatic mode. When temperature exceeds the fixed value, the temperature data is sent to Bluetooth module and is displayed to connected smartphone. While closing, the LED is set to blink indicating the system is running and “Waiting to CLOSE” is displayed on smartphone. When the curtain is fully closed and the close switch is engaged, “AUTO CLOSED” is displayed on smartphone.
else if (autoStat == 1 && tempC <= 32 && closeStat == HIGH && openClose == 0) // Temperature is 32 and below { measTemp(); HC05.println(tempC,1); // Send Temp data //HC05.println(“Waiting to OPEN”); //displays this when curtain opening do { blinkLED(); openStat = digitalRead(swOpen); digitalWrite(motFor, HIGH); digitalWrite(motRev, LOW); }while (openStat == LOW); // Wait until Open Sw = HIGH (Open switch click) digitalWrite(motFor, LOW); digitalWrite(ledOpen, HIGH); digitalWrite(ledClose, LOW); HC05.println(“Auto OPEN”); //displays this when curtain fully opened openClose = 1; } |
Figure 5.11: Curtain OPEN when temperature low (AUTO)
The above code initializes the curtain to open when the temperature goes below 32-degree Celsius in automatic mode. When temperature goes below the fixed value, the temperature data is sent to Bluetooth module and is displayed to connected smartphone. While opening, the LED is set to blink indicating the system is running and “Waiting to OPEN” is displayed on smartphone. When the curtain is fully opened and the open switch is engaged, “AUTO OPEN” is displayed on smartphone.
Cite This Work
To export a reference to this article please select a referencing stye below:
Related Services
View allDMCA / Removal Request
If you are the original writer of this essay and no longer wish to have your work published on UKEssays.com then please click the following link to email our support team:
Request essay removalRelated Services
Our academic writing and marking services can help you!
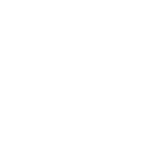
Freelance Writing Jobs
Looking for a flexible role?
Do you have a 2:1 degree or higher?
Study Resources
Free resources to assist you with your university studies!